6 Methods for Writing Unit Tests in Backend Development
Software Development Advice

6 Methods for Writing Unit Tests in Backend Development
Discovering robust strategies for unit testing can transform backend development, ensuring code reliability and maintainability. This guide explores tried-and-true methods that streamline the process of writing effective unit tests. From isolating external dependencies to handling edge cases, these techniques are designed to fortify backend code against future changes and errors.
- Use TDD for Backend Code
- Test Individual Functions
- Mock External Dependencies
- Test Edge Cases
- Test Positive and Negative Outcomes
- Write Concise and Readable Tests
Use TDD for Backend Code
My preferred method for writing unit tests for backend code involves using a test-driven development (TDD) approach, where I write tests before implementing the actual functionality. This ensures that the code is designed to meet specific requirements and helps catch bugs early in the development process. I usually rely on popular testing frameworks like **JUnit** for Java, **pytest** for Python, or **Mocha/Chai** for JavaScript/Node.js, depending on the language I'm working with. These frameworks offer powerful assertion libraries, easy test discovery, and integration with continuous integration (CI) tools, which streamline the testing process.
When structuring unit tests, I follow the **Arrange-Act-Assert (AAA)** pattern:
1. **Arrange**: Set up the necessary objects and prepare the environment.
2. **Act**: Execute the function or method being tested.
3. **Assert**: Verify that the output matches the expected result.
Additionally, I isolate units of code by using **mocks** or **stubs** for dependencies like databases, APIs, or services, ensuring that tests remain fast and focused solely on the logic being tested. This practice minimizes flakiness and makes it easier to pinpoint the source of errors.
Here's a simple example using **Python** with **pytest** to test a function that adds two numbers:
```python
# math_operations.py
def add(a, b):
return a + b
```
```python
# test_math_operations.py
from math_operations import add
def test_add():
# Arrange
num1 = 5
num2 = 3
# Act
result = add(num1, num2)
# Assert
assert result == 8
```
In this example, the test checks whether the `add()` function correctly sums two numbers. Running `pytest` will execute this test, and if the result matches the expected output (8), the test will pass. This simple approach scales well, allowing for more complex tests as the project grows.
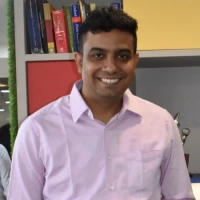
Test Individual Functions
Testing individual functions or methods allows for pinpointing specific issues within code execution. This method helps isolate problems and ensures each part of the code operates as intended. It results in detecting bugs more efficiently and maintaining a higher level of code quality.
This targeted approach also aids in simplifying the debugging process and makes the maintenance of code easier. Consider incorporating individual testing into your routine for effective code management.
Mock External Dependencies
Using mocks for external dependencies and services helps simulate interactions without relying on actual implementations. This practice ensures that tests are run in a controlled environment, eliminating the effects of variability in real services. Mocking also aids in identifying the behavior of code components when integrated with external systems.
This approach guarantees that the units under test perform correctly, independent of other services. To ensure your tests are robust, integrate mocks into your testing strategy.
Test Edge Cases
Testing edge cases and boundary conditions helps in anticipating unusual scenarios that may break the code. This technique ensures that the application handles unexpected inputs gracefully, reducing the likelihood of unexpected failures. By covering boundary conditions, the test suite becomes more comprehensive and reliable.
This method helps in creating a resilient and robust application. Make it a practice to consider edge cases during your testing phase.
Test Positive and Negative Outcomes
Testing both positive and negative outcomes validates the behavior of the code under all conditions. This ensures that the application not only functions correctly when expected inputs are provided but also responds appropriately to invalid or unexpected inputs. Testing both types of outcomes enhances the reliability and stability of the backend.
This comprehensive testing approach prevents unforeseen errors and supports smoother application performance. Start implementing tests for all possible outcomes in your testing plan.
Write Concise and Readable Tests
Producing concise, readable, and maintainable tests helps in making the testing process efficient and effective. Readable tests provide clear documentation for code functionality, which is essential for team collaboration. Maintainable tests ensure that as the code evolves, updating the test suite remains manageable.
Concise tests reduce complexity and make debugging easier. Focus on creating clear and maintainable test cases to improve the overall quality of your backend development.